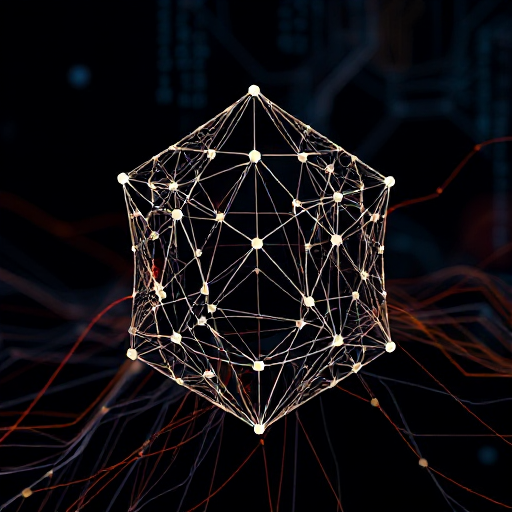
TensorFlow is one of the most popular open-source machine learning frameworks. Developed by Google, TensorFlow has been used to create models for deep learning, natural language processing, computer vision, and other domains of artificial intelligence. If you’re looking to get started with TensorFlow, this guide will walk you through the essential steps to help you learn it from scratch.
Table of Contents:
- Introduction to TensorFlow
- Why Learn TensorFlow?
- Prerequisites
- Setting Up Your Environment
- Basic Concepts in TensorFlow
- Hands-on Learning: Your First TensorFlow Program
- Working with Datasets
- Building Neural Networks with TensorFlow
- Advanced Topics
- Best Resources for Learning TensorFlow
- Conclusion
1. Introduction to TensorFlow
TensorFlow is a powerful framework for building and deploying machine learning models. It is a symbolic math library that allows you to define and train neural networks. TensorFlow was originally designed for deep learning applications but can be used for a variety of other tasks, including statistical modeling, time-series forecasting, and reinforcement learning.
At its core, TensorFlow provides an interface for defining computations that can be executed across various hardware platforms, including CPUs, GPUs, and TPUs (Tensor Processing Units).
2. Why Learn TensorFlow?
Learning TensorFlow offers several advantages:
- Industry Demand: TensorFlow is one of the most widely used frameworks in the machine learning and deep learning industries.
- Versatility: It can be used for a variety of machine learning tasks, from building simple linear regression models to complex deep neural networks.
- Scalability: TensorFlow is designed to work on a variety of platforms, from small mobile devices to large-scale distributed computing systems.
- Community Support: TensorFlow has a large and active community, making it easier to find solutions to problems and stay updated on the latest developments.
3. Prerequisites
Before diving into TensorFlow, there are a few foundational skills you should have:
- Python: TensorFlow is primarily a Python library, so familiarity with Python programming is essential.
- Mathematics: Basic knowledge of linear algebra, calculus, probability, and statistics will help you understand machine learning concepts, such as gradient descent and backpropagation.
- Machine Learning Basics: Understanding the fundamentals of machine learning, such as supervised and unsupervised learning, loss functions, and optimization, will give you a strong foundation.
4. Setting Up Your Environment
The first step in using TensorFlow is to set up your development environment. TensorFlow can be installed easily with Python’s package manager, pip.
Steps to Install TensorFlow:
- Install Python: Make sure you have Python 3.6 or later installed on your system.
Install pip: pip is a package manager that comes with Python. If it’s not installed, you can install it by running:
css
CopyEdit
python -m ensurepip –upgrade
Create a Virtual Environment (optional but recommended):
bash
CopyEdit
python -m venv tensorflow_env
source tensorflow_env/bin/activate # On Windows, use `tensorflow_env\Scripts\activate`
Install TensorFlow: Run the following command to install the latest version of TensorFlow:
CopyEdit
pip install tensorflow
Once installed, you can verify the installation by running:
python
CopyEdit
import tensorflow as tf
print(tf.__version__)
5. Basic Concepts in TensorFlow
To understand how TensorFlow works, you need to be familiar with some key concepts:
- Tensors: In TensorFlow, data is represented as tensors, which are multi-dimensional arrays. Tensors are the core data structure and can be manipulated to create machine learning models.
- Operations: Operations (Ops) are the building blocks of a TensorFlow graph. These are mathematical functions that perform computations on tensors, such as addition, multiplication, and matrix multiplication.
- Graphs: TensorFlow operations are defined as a graph. A graph consists of nodes (operations) and edges (data). The TensorFlow runtime uses this graph to execute operations in an optimized way.
- Sessions: In TensorFlow 1.x, computations were executed within a session. In TensorFlow 2.x, this is simplified, and you no longer need to explicitly use sessions.
6. Hands-on Learning: Your First TensorFlow Program
Let’s start by building a simple program in TensorFlow that performs a basic linear regression.
python
CopyEdit
import tensorflow as tf
import numpy as np
# Generate some synthetic data
X = np.array([1, 2, 3, 4], dtype=np.float32)
y = np.array([2, 4, 6, 8], dtype=np.float32)
# Define the model parameters
W = tf.Variable(0.0)
b = tf.Variable(0.0)
# Define the model’s prediction
def model(X):
return W * X + b
# Define the loss function (Mean Squared Error)
def loss_fn(y_pred, y_true):
return tf.reduce_mean(tf.square(y_pred – y_true))
# Optimizer (Gradient Descent)
optimizer = tf.optimizers.SGD(learning_rate=0.01)
# Training loop
for epoch in range(1000):
with tf.GradientTape() as tape:
y_pred = model(X)
loss = loss_fn(y_pred, y)
gradients = tape.gradient(loss, [W, b])
optimizer.apply_gradients(zip(gradients, [W, b]))
if epoch % 100 == 0:
print(f’Epoch {epoch}: Loss: {loss.numpy()}’)
print(f’Optimized W: {W.numpy()}, b: {b.numpy()}’)
7. Working with Datasets
Machine learning models rely heavily on datasets. TensorFlow provides several utilities to work with datasets, including tf.data, which allows you to load and preprocess large datasets efficiently.
Example:
python
CopyEdit
import tensorflow as tf
# Load a built-in dataset
(train_images, train_labels), (_, _) = tf.keras.datasets.fashion_mnist.load_data()
# Normalize the data
train_images = train_images / 255.0
# Create a TensorFlow dataset
train_dataset = tf.data.Dataset.from_tensor_slices((train_images, train_labels))
# Shuffle, batch, and prefetch for optimization
train_dataset = train_dataset.shuffle(60000).batch(32).prefetch(tf.data.experimental.AUTOTUNE)
8. Building Neural Networks with TensorFlow
TensorFlow has the Keras API, which makes building neural networks easy. Here’s an example of how to build a simple neural network for classifying images from the Fashion MNIST dataset.
python
CopyEdit
from tensorflow.keras import layers, models
# Build a Sequential model
model = models.Sequential([
layers.Flatten(input_shape=(28, 28)),
layers.Dense(128, activation=’relu’),
layers.Dropout(0.2),
layers.Dense(10)
])
# Compile the model
model.compile(optimizer=’adam’,
loss=tf.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=[‘accuracy’])
# Train the model
model.fit(train_images, train_labels, epochs=5)
9. Advanced Topics
Once you’re comfortable with the basics, you can explore more advanced topics in TensorFlow:
- Convolutional Neural Networks (CNNs): Used for image processing tasks.
- Recurrent Neural Networks (RNNs): Useful for sequential data, such as time series and natural language.
- Transfer Learning: Fine-tuning pre-trained models for specific tasks.
- TensorFlow Lite: Deploying models to mobile and embedded devices.
- TensorFlow.js: Running TensorFlow models in the browser.
10. Best Resources for Learning TensorFlow
Here are some excellent resources for learning TensorFlow:
- Official TensorFlow Documentation: https://www.tensorflow.org/
- Coursera – Deep Learning Specialization by Andrew Ng: A great introduction to deep learning.
- TensorFlow YouTube Channel: Provides tutorials and talks on various TensorFlow topics.
- Books:
- “Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow” by Aurélien Géron.
- “Deep Learning with Python” by François Chollet.
Learning TensorFlow from scratch can be a rewarding experience, especially with the wide range of tools and resources available. Start with the fundamentals, build your first models, and gradually progress to more complex architectures. The key to mastering TensorFlow is consistent practice, learning from others, and experimenting with different models and datasets.
By following this guide, you’ll have a solid foundation in TensorFlow, allowing you to build powerful machine learning models and apply them to real-world problems.