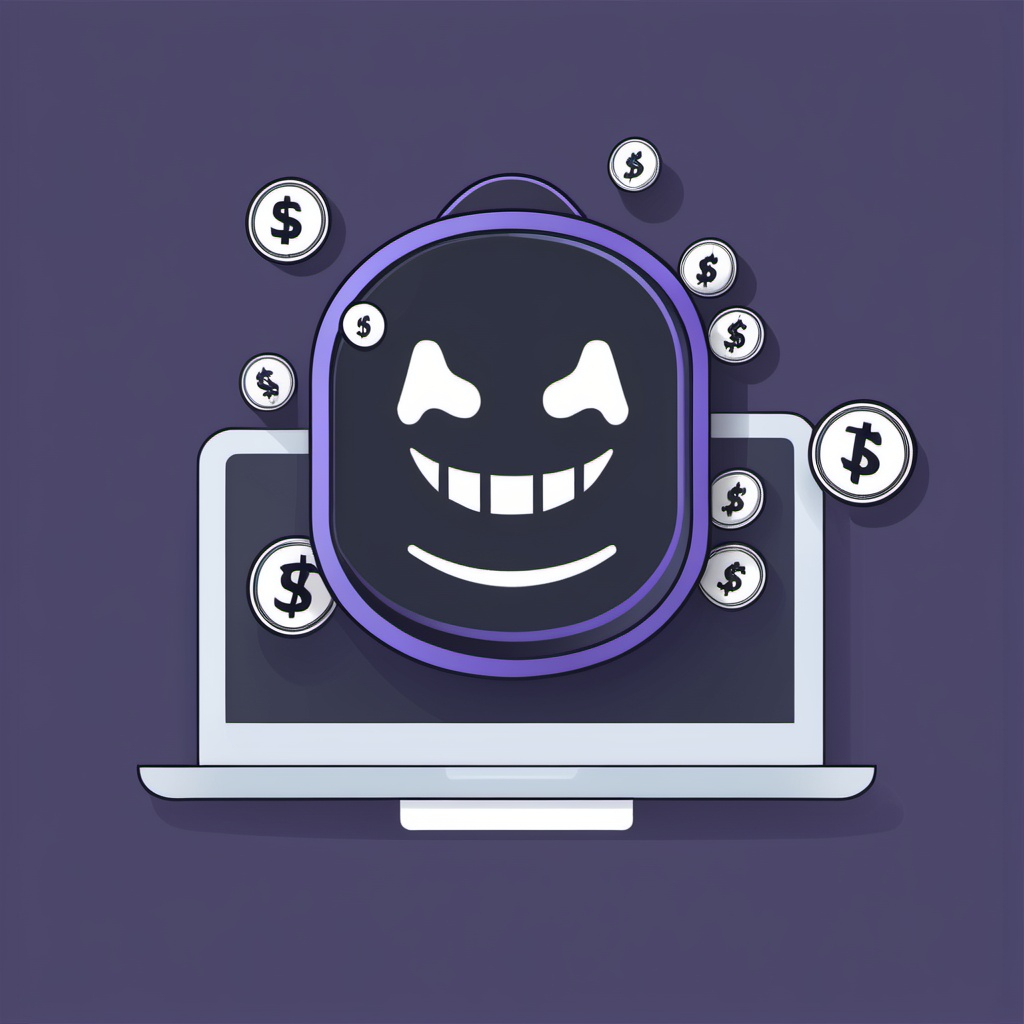
Docker is one of the most powerful tools in modern software development, providing a way to package applications and their dependencies into containers. These containers are lightweight, portable, and easy to deploy across various environments. As a developer, IT professional, or DevOps engineer, mastering Docker can significantly streamline your workflows and improve your efficiency. In this article, we’ll explore the best way to learn Docker, breaking down the learning process into manageable steps, useful resources, and practical tips.
1. Understand the Basics of Docker
Before diving into Docker, it is important to have a basic understanding of a few concepts that form the foundation of containerization. Some essential concepts to familiarize yourself with include:
- Containers: A container is a standardized unit of software that packages an application and all its dependencies into a single, portable package. This includes the code, libraries, configurations, and runtime.
- Images: Docker images are the blueprints for containers. They contain the application and all the necessary components to run it. Images are built from Dockerfiles, which define how the image should be constructed.
- Docker Engine: This is the core component of Docker that runs containers. It consists of the Docker Daemon, the Docker CLI (Command Line Interface), and the Docker API.
- Docker Hub: Docker Hub is a public registry where developers can share and download Docker images. It’s a great place to start when searching for pre-configured images to use in your projects.
Familiarizing yourself with these terms will lay a strong foundation for understanding Docker.
2. Set Up Docker on Your Machine
The first hands-on step in learning Docker is to set it up on your system. Docker supports various operating systems, including Windows, macOS, and Linux. You can follow the official Docker installation guides for each platform:
- For Windows and Mac: Docker Desktop provides an easy-to-use interface for managing Docker containers.
- For Linux: You can install Docker through your distribution’s package manager (e.g., apt for Ubuntu, yum for CentOS).
Once Docker is installed, verify the installation by running the command:
bash
Copy code
docker –version
This should display the installed Docker version, confirming that the setup was successful.
3. Start with Basic Commands
After setting up Docker, it’s time to start learning the basic commands that form the core of Docker’s functionality. Start by running a simple container with the following command:
bash
Copy code
docker run hello-world
This will download a test image from Docker Hub and run it inside a container. This is a great way to confirm that Docker is working correctly.
Some other fundamental commands to familiarize yourself with include:
- docker ps: Lists all running containers.
- docker images: Lists all available images on your system.
- docker run <image_name>: Runs a container from a specific image.
- docker stop <container_id>: Stops a running container.
- docker rm <container_id>: Removes a container.
- docker rmi <image_name>: Removes an image from the local system.
4. Learn Dockerfiles and Building Images
As you begin to work with Docker, you’ll quickly realize that creating custom containers is essential. This is where Dockerfiles come in. A Dockerfile is a script that contains instructions for building a Docker image.
A basic Dockerfile might look like this:
dockerfile
Copy code
# Use an official Python runtime as the base image
FROM python:3.8-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install –no-cache-dir -r requirements.txt
# Make port 80 available to the world outside the container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD [“python”, “app.py”]
This Dockerfile sets up a Python environment, installs dependencies, exposes a port, and specifies the command to run when the container starts. You can build an image from the Dockerfile with the following command:
bash
Copy code
docker build -t my-python-app .
This process will give you a deeper understanding of how Docker images are constructed and configured.
5. Practice with Real Projects
Hands-on practice is crucial for mastering Docker. Start applying what you’ve learned by containerizing real applications. Here are a few ideas for beginner projects:
- Web Server: Set up a basic web server (e.g., using Nginx or Apache) inside a Docker container.
- Database Containers: Learn to containerize databases like MySQL, PostgreSQL, or MongoDB. Create a multi-container application that includes both the database and the web server.
- Microservices: Docker shines in microservices architectures. Try containerizing multiple microservices that interact with each other and deploy them using Docker Compose.
By working on real-world applications, you’ll gain practical experience in building, running, and managing containers.
6. Explore Docker Compose for Multi-Container Applications
When you need to work with multiple containers that need to interact with each other, Docker Compose is an essential tool. Docker Compose allows you to define and manage multi-container applications with a simple YAML configuration file (docker-compose.yml).
Here’s a simple example of a docker-compose.yml file:
yaml
Copy code
version: ‘3’
services:
web:
image: nginx:latest
ports:
– “80:80”
app:
image: my-app
depends_on:
– web
In this example, Docker Compose runs both a web server (Nginx) and an app container. You can start both containers with a single command:
bash
Copy code
docker-compose up
Learning Docker Compose will help you manage more complex applications and understand how different containers can work together.
7. Learn Docker Networking
Docker provides several networking options that allow containers to communicate with each other and the outside world. Understanding Docker’s network model will help you design scalable and secure containerized applications.
- Bridge Network: The default network for containers, allowing communication between containers on the same host.
- Host Network: The container shares the host’s network stack.
- Overlay Network: Used for communication between containers on different hosts, typically in a Docker Swarm or Kubernetes setup.
Experiment with different network modes to understand how containers interact in different environments.
8. Master Docker Volumes for Data Persistence
By default, data inside containers is ephemeral—meaning it is lost when the container stops or is removed. Docker volumes provide a way to persist data across container restarts.
You can create a volume with:
bash
Copy code
docker volume create my-volume
You can then mount the volume to a container to store data persistently:
bash
Copy code
docker run -v my-volume:/data my-app
Learning how to use volumes effectively is essential when building applications that require persistent data storage.
9. Learn Docker Swarm and Kubernetes for Orchestration
As your use of Docker grows, you may want to explore container orchestration tools like Docker Swarm and Kubernetes. These tools allow you to manage clusters of containers and deploy applications at scale.
- Docker Swarm is Docker’s native clustering tool. It enables you to deploy multi-container applications and manage them across a cluster of machines.
- Kubernetes is the industry-standard container orchestration platform. While more complex than Docker Swarm, Kubernetes offers powerful features for managing containers in production environments.
Start by learning Docker Swarm, as it integrates seamlessly with Docker. Once comfortable, move on to Kubernetes to handle larger-scale applications.
10. Stay Updated and Join the Docker Community
Docker is a rapidly evolving technology, and staying up to date is key. Join the Docker community through forums, blogs, and events like DockerCon to keep learning. Follow Docker’s official documentation, tutorials, and YouTube channels for the latest features and best practices.
You can also learn a lot from open-source projects and repositories on GitHub, where many developers share their Docker-related projects.
Learning Docker is an exciting journey that opens up numerous possibilities for modern software development. By starting with the basics, setting up Docker on your system, mastering commands, and working on real projects, you will gain the practical experience needed to become proficient. As you progress, dive into advanced topics like Docker Compose, networking, volumes, and container orchestration. With consistent practice and engagement with the Docker community, you’ll be well on your way to becoming a Docker expert.
Happy learning, and enjoy the process of exploring this powerful tool!